How Developers Can Improve Their Debugging Competencies By Gustavo Woltmann
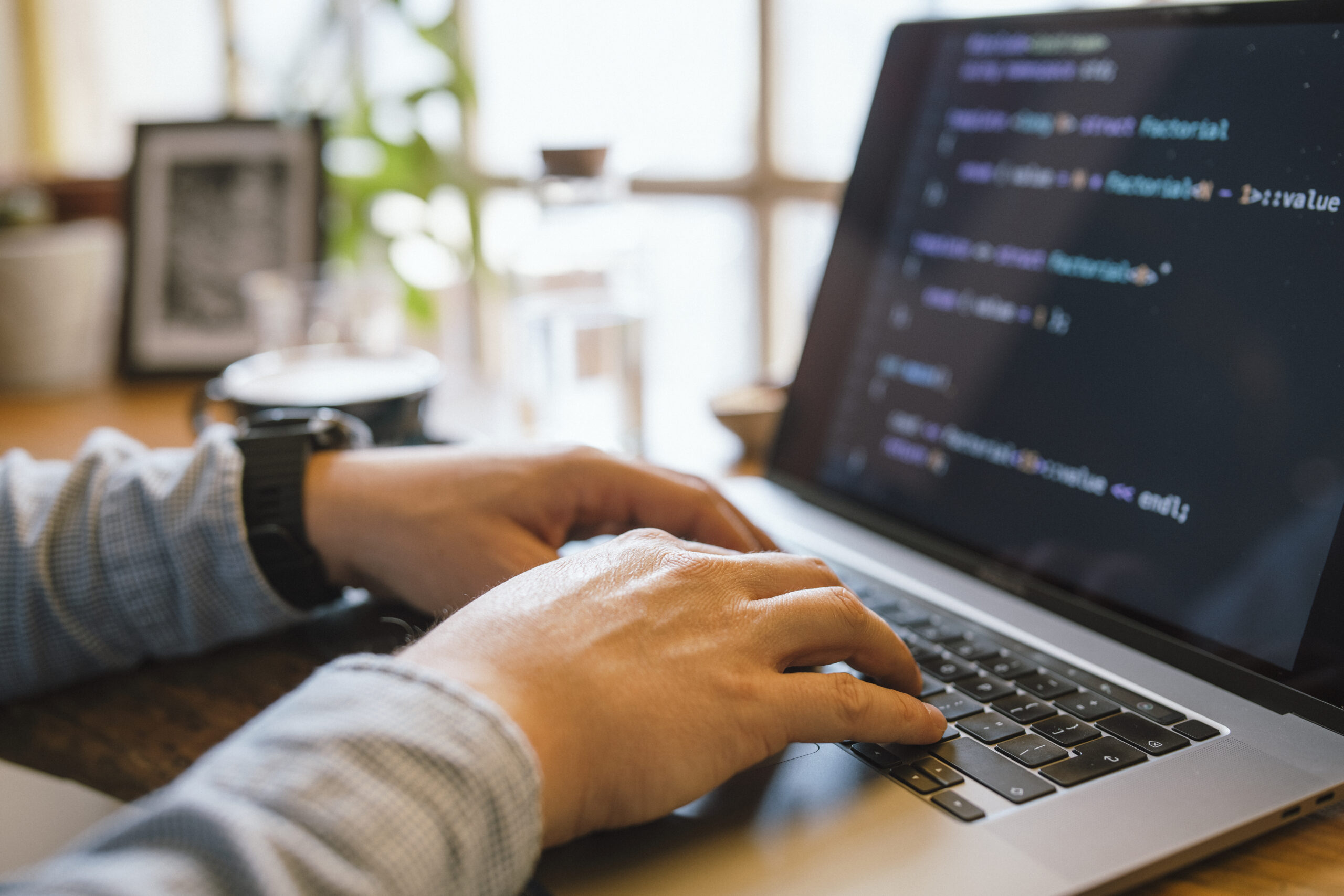
Debugging is Among the most crucial — nonetheless frequently disregarded — competencies in a developer’s toolkit. It isn't nearly repairing damaged code; it’s about being familiar with how and why items go Completely wrong, and learning to Feel methodically to resolve issues proficiently. Whether you're a rookie or even a seasoned developer, sharpening your debugging abilities can save several hours of aggravation and significantly enhance your productivity. Listed below are many tactics that will help builders stage up their debugging video game by me, Gustavo Woltmann.
Grasp Your Instruments
Among the list of fastest approaches developers can elevate their debugging capabilities is by mastering the resources they use each day. While crafting code is one Element of progress, being aware of the way to communicate with it efficiently throughout execution is Similarly significant. Modern-day growth environments appear equipped with impressive debugging abilities — but several developers only scratch the area of what these instruments can do.
Consider, for instance, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment help you set breakpoints, inspect the value of variables at runtime, action by means of code line by line, and even modify code over the fly. When utilised effectively, they Enable you to observe particularly how your code behaves for the duration of execution, that is a must have for tracking down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-conclusion developers. They enable you to inspect the DOM, monitor community requests, view true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can convert irritating UI troubles into manageable duties.
For backend or procedure-degree builders, equipment like GDB (GNU Debugger), Valgrind, or LLDB offer deep Management about operating procedures and memory administration. Understanding these applications might have a steeper Discovering curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn into snug with version Handle devices like Git to be familiar with code background, locate the precise minute bugs were being released, and isolate problematic modifications.
Eventually, mastering your instruments usually means likely further than default settings and shortcuts — it’s about building an intimate familiarity with your enhancement environment to ensure that when concerns occur, you’re not missing at the hours of darkness. The greater you are aware of your tools, the more time you'll be able to devote fixing the actual difficulty as an alternative to fumbling by way of the method.
Reproduce the challenge
Among the most important — and infrequently forgotten — techniques in successful debugging is reproducing the challenge. Ahead of jumping to the code or generating guesses, developers need to have to make a constant atmosphere or situation exactly where the bug reliably appears. Without reproducibility, correcting a bug turns into a sport of chance, usually resulting in wasted time and fragile code modifications.
The initial step in reproducing a dilemma is collecting as much context as you possibly can. Inquire questions like: What steps triggered The problem? Which surroundings was it in — growth, staging, or generation? Are there any logs, screenshots, or error messages? The more depth you might have, the less difficult it turns into to isolate the exact conditions less than which the bug happens.
When you’ve collected plenty of details, try to recreate the situation in your local natural environment. This could necessarily mean inputting precisely the same data, simulating related user interactions, or mimicking process states. If the issue seems intermittently, consider creating automatic exams that replicate the sting cases or condition transitions associated. These exams don't just aid expose the condition but additionally protect against regressions in the future.
At times, The difficulty might be setting-unique — it might come about only on sure working programs, browsers, or under certain configurations. Working with applications like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is usually instrumental in replicating this kind of bugs.
Reproducing the trouble isn’t merely a move — it’s a state of mind. It demands persistence, observation, plus a methodical solution. But once you can constantly recreate the bug, you are previously midway to correcting it. Which has a reproducible scenario, You can utilize your debugging equipment far more correctly, examination probable fixes properly, and connect extra Evidently with your team or customers. It turns an summary grievance right into a concrete problem — Which’s where developers prosper.
Browse and Understand the Mistake Messages
Mistake messages are often the most valuable clues a developer has when something goes Completely wrong. Rather then looking at them as discouraging interruptions, developers must discover to treat mistake messages as immediate communications from your procedure. They typically let you know precisely what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start off by reading through the concept very carefully As well as in whole. A lot of developers, specially when beneath time pressure, look at the primary line and instantly get started generating assumptions. But deeper from the error stack or logs may perhaps lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like yahoo — read and fully grasp them initial.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it place to a specific file and line selection? What module or purpose triggered it? These inquiries can guide your investigation and position you toward the accountable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Understanding to acknowledge these can significantly accelerate your debugging system.
Some mistakes are obscure or generic, As well as in These situations, it’s very important to examine the context through which the mistake happened. Verify relevant log entries, enter values, and up to date modifications while in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger concerns and supply hints about probable bugs.
Finally, error messages usually are not your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, helping you pinpoint problems more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Just about the most strong instruments in a very developer’s debugging toolkit. When made use of correctly, it offers serious-time insights into how an software behaves, encouraging you understand click here what’s going on underneath the hood without having to pause execution or move in the code line by line.
A very good logging strategy starts with knowing what to log and at what level. Popular logging concentrations involve DEBUG, Facts, Alert, Mistake, and Lethal. Use DEBUG for specific diagnostic facts all through progress, Details for standard functions (like productive begin-ups), WARN for opportunity difficulties that don’t split the application, ERROR for precise challenges, and Deadly when the system can’t go on.
Prevent flooding your logs with extreme or irrelevant info. An excessive amount of logging can obscure important messages and decelerate your program. Concentrate on crucial occasions, point out alterations, input/output values, and important determination points in the code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace problems in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments in which stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about equilibrium and clarity. Having a very well-believed-out logging technique, you can decrease the time it will require to spot concerns, attain deeper visibility into your programs, and Enhance the In general maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To efficiently discover and take care of bugs, developers should technique the procedure similar to a detective resolving a secret. This mindset aids break down elaborate issues into manageable elements and observe clues logically to uncover the root result in.
Start off by accumulating evidence. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or performance problems. Much like a detective surveys a crime scene, gather as much pertinent details as you may without the need of leaping to conclusions. Use logs, exam scenarios, and person stories to piece jointly a clear image of what’s taking place.
Subsequent, type hypotheses. Inquire yourself: What could be causing this actions? Have any variations a short while ago been designed to your codebase? Has this situation transpired just before below comparable circumstances? The goal should be to slender down opportunities and recognize possible culprits.
Then, test your theories systematically. Seek to recreate the situation in the controlled environment. For those who suspect a certain perform or component, isolate it and validate if the issue persists. Similar to a detective conducting interviews, check with your code queries and let the final results direct you nearer to the truth.
Pay near awareness to tiny particulars. Bugs often cover inside the least predicted locations—similar to a missing semicolon, an off-by-one mistake, or even a race condition. Be extensive and affected person, resisting the urge to patch The difficulty with out absolutely comprehension it. Temporary fixes may possibly hide the true issue, just for it to resurface later.
And finally, keep notes on Whatever you experimented with and acquired. Just as detectives log their investigations, documenting your debugging system can conserve time for long run issues and support Many others realize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach troubles methodically, and become more effective at uncovering concealed challenges in sophisticated programs.
Generate Tests
Creating assessments is among the simplest ways to boost your debugging capabilities and In general development efficiency. Exams not merely help catch bugs early but additionally function a security Internet that offers you assurance when making modifications in your codebase. A effectively-examined application is simpler to debug as it means that you can pinpoint particularly where by and when a problem occurs.
Get started with device checks, which deal with unique capabilities or modules. These compact, isolated checks can immediately expose no matter if a certain bit of logic is Doing work as anticipated. Whenever a check fails, you instantly know exactly where to look, significantly reducing some time expended debugging. Unit tests are especially practical for catching regression bugs—challenges that reappear immediately after Earlier getting set.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These enable be certain that different parts of your software operate with each other effortlessly. They’re specially beneficial for catching bugs that occur in elaborate programs with numerous factors or companies interacting. If some thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating checks also forces you to Imagine critically about your code. To check a characteristic properly, you require to know its inputs, envisioned outputs, and edge instances. This degree of being familiar with By natural means potential customers to higher code composition and fewer bugs.
When debugging a concern, crafting a failing examination that reproduces the bug is usually a powerful initial step. Once the examination fails continuously, you'll be able to center on fixing the bug and look at your exam pass when The problem is solved. This approach makes sure that the exact same bug doesn’t return in the future.
In brief, producing checks turns debugging from a aggravating guessing video game right into a structured and predictable process—aiding you capture extra bugs, faster and even more reliably.
Just take Breaks
When debugging a tough difficulty, it’s simple to become immersed in the challenge—observing your monitor for hours, making an attempt Resolution immediately after Alternative. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen annoyance, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for way too very long, cognitive tiredness sets in. You would possibly start out overlooking evident glitches or misreading code you wrote just several hours previously. In this particular condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee break, or even switching to another undertaking for ten–15 minutes can refresh your concentrate. Many builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially in the course of lengthier debugging classes. Sitting in front of a monitor, mentally caught, is not only unproductive but will also draining. Stepping away enables you to return with renewed energy in addition to a clearer way of thinking. You could all of a sudden discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
For those who’re caught, a good guideline is to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment split. Use that point to move all over, stretch, or do a thing unrelated to code. It might experience counterintuitive, Specifically less than restricted deadlines, but it really truly causes a lot quicker and simpler debugging Ultimately.
In brief, getting breaks isn't an indication of weak spot—it’s a smart approach. It presents your brain Place to breathe, increases your viewpoint, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Find out From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It truly is a possibility to grow as being a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can train you a little something valuable should you make time to replicate and review what went wrong.
Begin by asking by yourself some vital questions once the bug is resolved: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code opinions, or logging? The solutions generally expose blind places with your workflow or comprehension and make it easier to Make more robust coding behaviors transferring ahead.
Documenting bugs can be a fantastic routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and Anything you figured out. After some time, you’ll start to see patterns—recurring issues or common issues—you could proactively prevent.
In crew environments, sharing Everything you've discovered from the bug with the peers may be especially impressive. No matter if it’s by way of a Slack message, a brief compose-up, or a quick know-how-sharing session, aiding Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who generate excellent code, but those who continually learn from their problems.
In the end, Every single bug you fix adds a completely new layer for your talent established. So up coming time you squash a bug, have a moment to mirror—you’ll appear absent a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging abilities normally takes time, observe, and persistence — even so the payoff is large. It tends to make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.